Pointers are optimized away or translated to readable Java classes, function pointers are translated to method references. Full support for unsigned types. Full support for goto statements. Full support for native libraries; the translated Java project seamlessly interfaces with libraries (GMP, Ncurses, X11.) the C project used. The Java properties indicate the location of the jar file, the name of the Main class, and the version of the JRE to be used. The other properties include a pointer to the icon file and to the Java application stub file that is the native executable. Creating Mac OS X Java Applications on Other Platforms.
- Running the command: $ gcc -S geeks.c This will cause gcc to run the compiler, generating an assembly file geeks.s, and go no further.(Normally it would then invoke the assembler to generate an object- code file.).
- Convert binary data into java or c sourcecode for easy integration in your project, with the free sfk bin-to-src command for Windows, Mac OS X, Linux and Raspberry Pi. download the free Swiss File Knife Base from Sourceforge. open the Windows CMD command line, Mac OS X Terminal or Linux shell.
Note: we recommend to have a proper knowledge of OSX and Java. We’re not going to explain how to do certain things, because the article would be too long.
One of the most difficult tasks while developing Crazy Belts has been setting the game to OSX. There is little information and very few tools to do it; in fact, only one tool available to pack a jar file into an app. This was a mandatory step to publish our game inside and outside OSX App Store. In Jemchicomac, we used two process for this:
- Gatekeeper: to publish outside the store
- Sandbox: to publish inside the store
Gatekeeper prevents third party apps to be run in the system without Apple’s signature and verification. It’s conceived for those apps that come from another stores or websites not related to the App Store. User can disable Gatekeeper, but we consider that’s fair to demonstrate you’re a valid partner of Apple and you app is trustworthy.
On the other hand, Sandbox is a tool that encapsulates you app and check it: if it’s harmful, then Sandbox aparts it from the rest of the operative system. This is an essential requirement to publish in the Mac Store and it has a different signature than Gatekeeper.
Before all of this, as you have imagined, it’s necessary to convert our jar in an app, whatever our commercial goal in OSX is. We need the following tools:
- App Bundler: https://bitbucket.org/infinitekind/appbundler, that’s going to convert our jar to app. You have to download it, compile and pack it with ant. You must do it in Mac, not Windows.
- JRE or JDK: you need Java in your computer. It doesn’t have to be Oracle’s Java Virtual Machine, but it’s the one we used.
- Mac Developer account: essential to sign the app.
Given those ingredients, we can start creating our app. We need Java 7 (in our OSX system, we find only Java 6 as preinstalled). Once we install our JVM with Java 7, we must go to one of the jdk or jre installations. For instance: …/home/Library/Java/JavaVirtualMachines/jdk1.7.0_51.jdk. We click on the icon, and if the active version of Java is SE6 we have to disable it , and check SE7.
Now you have to configure Java Home: http://www.mkyong.com/java/how-to-set-java_home-environment-variable-on-mac-os-x/
Once all of this is done, we’re ready for the next step. We create a folder with all necessary thing to build our app (I called mine “build”).
- A build.xml file with the information for Ant of Java to create our app
- An empty info.plist file. We just create a file called this.
- A folder containing appbundler -1.0ea.jar.
- A dist folder with the jar file we want to convert.
- Optionally, a icns file that we have previously worked in and contains a whole family of icons with different sizes, to embellish the app file.
Before going on, we have to warn you that all this work is made with the Terminal and we have to go to each directory when required. For instance, if my build folder is at the Desktop, in the Terminal I must go using
[bash]cd desktop/build.[/bash]For me, build.xml is like this:
[xml]<?xml version='1.0' encoding='UTF-8'?>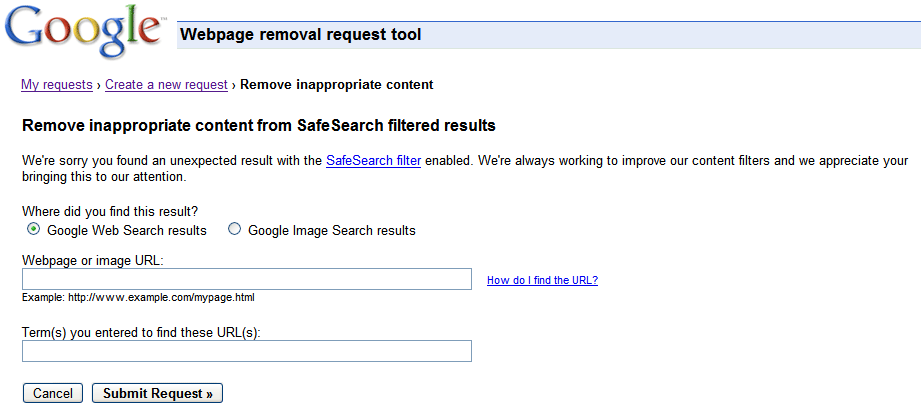
<project name='CrazyBelts' default='default' basedir='.'>
<target name='bundle'>
<taskdef name='bundleapp'
classpath='lib/appbundler-1.0ea.jar'

classname='com.oracle.appbundler.AppBundlerTask'/>
<bundleapp
outputdirectory='dist'
name='Crazy Belts'
displayname='Crazy Belts'
identifier='com.company.crazybelts'
shortversion='1.3.0'
version='1.3.0'
icon='icon.icns'
mainclassname='org.eclipse.jdt.internal.jarinjarloader.JarRsrcLoader'
copyright='2014 Company'
applicationCategory='public.app-category.puzzle-games'>
<classpath file='dist/CrazyBelts12FINAL.jar'/>
<runtime/>
<!– Workaround since the icon parameter for bundleapp doesn’t work –>
<option value='-Xdock:icon=Contents/Resources/${bundle.icon}'/>
<arch name='x86_64'/>
<arch name='i386'/>
</bundleapp>
</target>
</project>[/xml]
Let’see each entry:
[xml]<project name='CrazyBelts' default='default' basedir='.'>[/xml]We must change the name to our app name.
[xml]<target name='bundle'>[/xml]This is the name of our Ant task, meaning this is the one we should use when calling for Ant. In our example, once we’re set in the directory we type:
[bash]ant bundle[/bash]Taskdef must match the label of the task we want to run. In classpath we type the directory where the AppBundler is located. Finally, we leave classname without changes.
[xml]<bundleappoutputdirectory='dist'
name='Crazy Belts'
displayname='Crazy Belts'
identifier='com.company.crazybelts'
shortversion='1.3.0'
version='1.3.0'
icon='icon.icns'
mainclassname='org.eclipse.jdt.internal.jarinjarloader.JarRsrcLoader'
copyright='2014 Company'
applicationCategory='public.app-category.puzzle-games'>[/xml]
The main block of build.xml:
- Output directory: we can put here the jar to pack (in our case, we did this), and it’ll also contain the app when it’s created.
- name: the name of the app
- display name: the name as displayed

- identifier: it must match an App ID registered in your Mac Developer account. This registration can be done afterwards, and it’s only necessary if you’re going to distribute in the Mac Store, not for third parties.
- Shortversion: the game version
- Icon: this is the icon we mentioned before, in the root of the build folder.
- Mainclassname: this is a very important entry. We must indicate the class that runs the app. In our case, we used Eclipse and we did spend so much time setting the main class of the app, while this IDE actually used his own Leader class when creating the exe jar.
- Copyright: our national copyright
- applicationCategory: our app category code. Visit this link to know more about it: https://developer.apple.com/library/Mac/releasenotes/General/SubmittingToMacAppStore/#//apple_ref/doc/uid/TP40010572-CH16-SW8
<!– Workaround since the icon parameter for bundleapp doesn’t work –>
<option value='-Xdock:icon=Contents/Resources/${bundle.icon}'/>
<arch name='x86_64'/>
<arch name='i386'/>[/xml]
Only runtime.dir is important for us, we don’t touch the rest. This entry is optional and if we don’t include it in our app it won’t have an embedded jdk. Good news are that doesn’t require much space; bad news are that if the user doesn’t have the correct jdk or jre, the app won’t run, so it’s poorly advisable. This includes the path where our jdk is located; if we don’t know it and we’re using Eclipse, we can go to Preferences and check the jre.
After that, we must go to the Terminal and type:
[bash]ant bundle.[/bash]A package with .app extension will be created, and it will contain our .jar
The final part is signing the app. In our example, we must use another way apart from Xcode. Everything will be done using Terminal.
We’re going to start with the Gatekeeper version, the easier one. To sign an app and be accepted by this technology we must create a certificate with a Team Agent account. Be very careful with this: to publish outside the App Store you must create a Developer ID Application Certificate or Developer ID Installer Certificate, depending on whether we want to only sign the app as it is or to pack it into an installer (that also has to be signed).
To create a certificate, we always have to go to the development console in the section “Certificates, Identifiers & Profiles”. Once created and added to the Keychain (check carefully we have the private key associated to the public one), we just set into the app directory and type:
[bash]codesign -v -f -s 'Developer ID Application' 'myapp.app'[/bash]If we get an error message, we type the following command: export CODESIGN_ALLOCATE=”/Applications/Xcode.app/Contents/Developer/usr/bin/codesign_allocate”
And if everything goes right, we must see this: signed bundle with Mach-O universal (i386 x86_64) [com.company.myapp]
There are many differences and similarities between the C++ programming language and Java. A list of top differences between C++ and Java are given below:
Comparison Index | C++ | Java |
---|---|---|
Platform-independent | C++ is platform-dependent. | Java is platform-independent. |
Mainly used for | C++ is mainly used for system programming. | Java is mainly used for application programming. It is widely used in window, web-based, enterprise and mobile applications. |
Design Goal | C++ was designed for systems and applications programming. It was an extension of C programming language. | Java was designed and created as an interpreter for printing systems but later extended as a support network computing. It was designed with a goal of being easy to use and accessible to a broader audience. |
Goto | C++ supports the goto statement. | Java doesn't support the goto statement. |
Multiple inheritance | C++ supports multiple inheritance. | Java doesn't support multiple inheritance through class. It can be achieved by interfaces in java. |
Operator Overloading | C++ supports operator overloading. | Java doesn't support operator overloading. |
Pointers | C++ supports pointers. You can write pointer program in C++. | Java supports pointer internally. However, you can't write the pointer program in java. It means java has restricted pointer support in java. |
Compiler and Interpreter | C++ uses compiler only. C++ is compiled and run using the compiler which converts source code into machine code so, C++ is platform dependent. | Java uses compiler and interpreter both. Java source code is converted into bytecode at compilation time. The interpreter executes this bytecode at runtime and produces output. Java is interpreted that is why it is platform independent. |
Call by Value and Call by reference | C++ supports both call by value and call by reference. | Java supports call by value only. There is no call by reference in java. |
Structure and Union | C++ supports structures and unions. | Java doesn't support structures and unions. |
Thread Support | C++ doesn't have built-in support for threads. It relies on third-party libraries for thread support. | Java has built-in thread support. |
Documentation comment | C++ doesn't support documentation comment. | Java supports documentation comment (/** ... */) to create documentation for java source code. |
Virtual Keyword | C++ supports virtual keyword so that we can decide whether or not override a function. | Java has no virtual keyword. We can override all non-static methods by default. In other words, non-static methods are virtual by default. |
unsigned right shift >>> | C++ doesn't support >>> operator. | Java supports unsigned right shift >>> operator that fills zero at the top for the negative numbers. For positive numbers, it works same like >> operator. |
Inheritance Tree | C++ creates a new inheritance tree always. | Java uses a single inheritance tree always because all classes are the child of Object class in java. The object class is the root of the inheritance tree in java. |
Hardware | C++ is nearer to hardware. | Java is not so interactive with hardware. |
Object-oriented | C++ is an object-oriented language. However, in C language, single root hierarchy is not possible. | Java is also an object-oriented language. However, everything (except fundamental types) is an object in Java. It is a single root hierarchy as everything gets derived from java.lang.Object. |
Note
- Java doesn't support default arguments like C++.
- Java does not support header files like C++. Java uses the import keyword to include different classes and methods.
C++ Example
File: main.cpp
Java Example
C 2b 2b To Java Converter For Mac 64-bit
File: Simple.java